JavaScript DOM access
When we created our first page with HTML and loaded it onto a browser, the browser further converted the HTML into something called the DOM (document object model). This DOM then allows languages such as JavaScript to access and manipulate the structure and contents of the HTML!
Thus, in this page, we will cover the following ways with which we can use JavaScript to access HTML from a webpage, through the DOM:
- The
document
object - The
getElement...
methods - The
querySelector
method - The
querySelectorAll
method - DOM traversal
parentNode
propertychildren
propertyfirstChild
property
The document
object
In the JavaScript console, we can access the current webpage's DOM (i.e. a JavaScript-compatible representation of HTML) with the document
object:
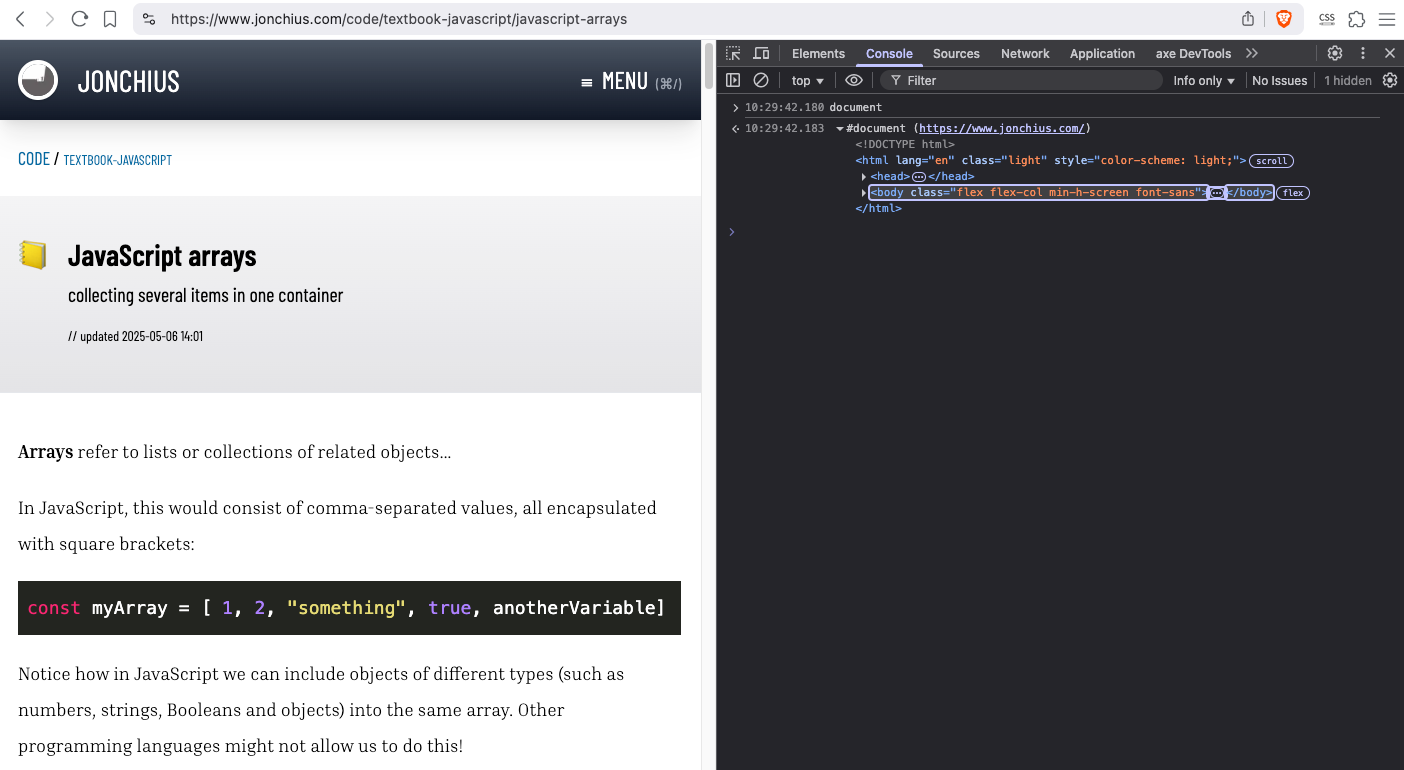
The getElement...
methods
With the document
object, we can then access the DOM elements (i.e. HTML tags) with other built-in methods such as:
getElementById(...)
getElementsByClassName(...)
getElementsByTagName(...)
Note that when using these methods, we would access (the DOM representation of) the HTML tags and not the content of those tags. To access the content, we will later look at other built-in methods.
getElementById(...)
The getElementById(myId)
built-in method returns an Element
object, for the HTML tag that has an id
attribute value of myId
...
Confused? Let's look at an example:
<html>
<head></head>
<body>
<h1>Heading 1</h1>
<h2 id="myHeading">Heading 2a</h2>
<h2>Heading 2b</h2>
</body>
</html>
We can then use getElementById(...)
to access the tag with some passed-in id
value, in this case, myHeading
:
let myH2 = document.getElementById('myHeading')
console.log(myH2)
// this will print out an Element object
An example with an actual webpage, where the id
is head-branding
:
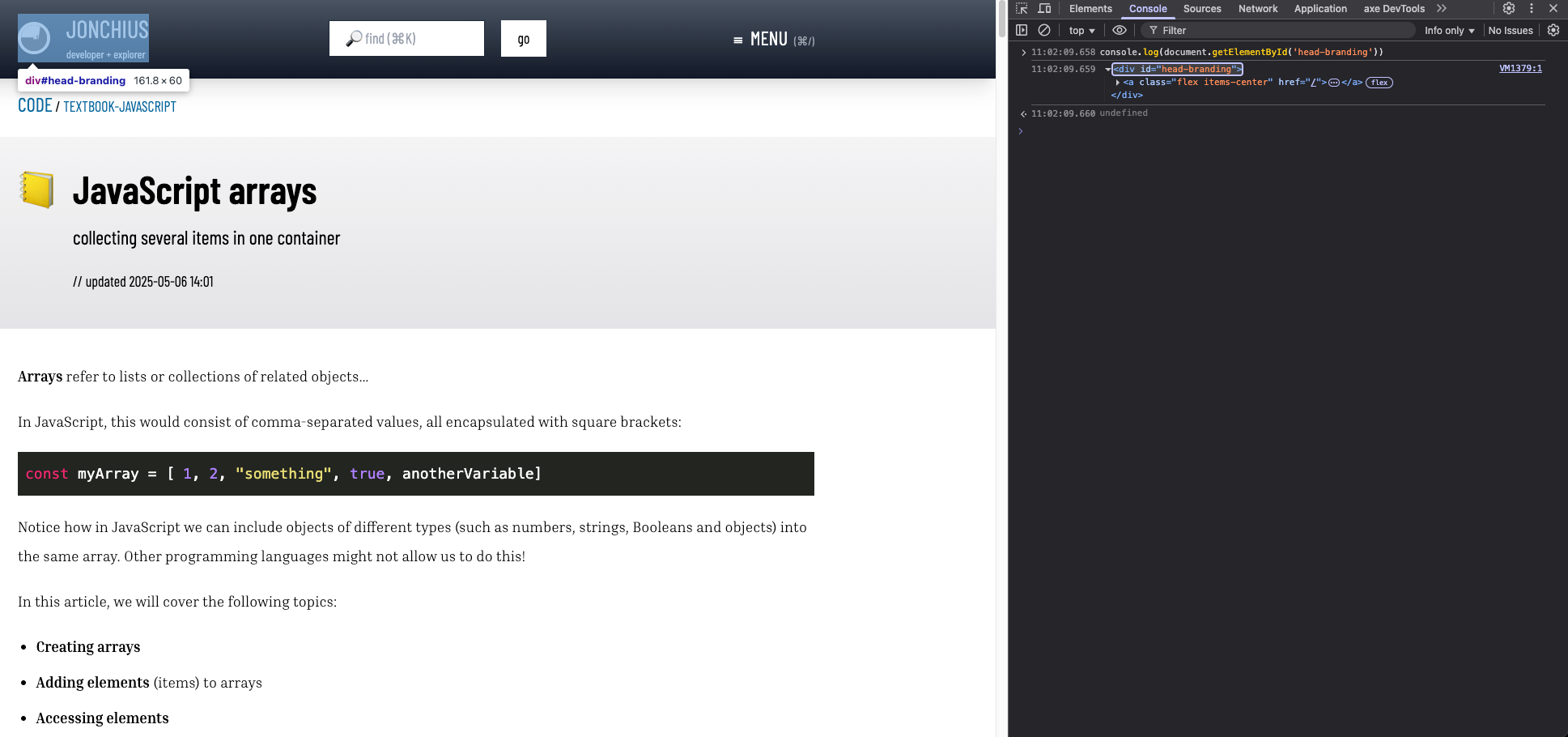
getElementsByClassName(...)
The getElementsByClassName(myClassName)
built-in method returns an HTMLCollection
of any element(s) with a class
attribute value of myClassName
:
<html>
<head></head>
<body>
<h1 class="myClass">Heading 1</h1>
<h2 class="myClass">Heading 2a</h2>
<h2>Heading 2b</h2>
</body>
</html>
We can then use getElementsByClassName(myClassName)
to access any tags with any class name:
let myClassTags = document.getElementsByClassName('myClass')
console.log(myClass)
// this will print out an HTMLCollection object
We can even gather tags that have one of many class names:
let tagsWithMyClasses = document.getElementsByClassName('myClass1 myClass2 myClass3')
console.log(tagsWithMyClasses)
// this will print out an HTMLCollection object
getElementsByTagName(...)
Similar to getElementsByClassName(...)
, the getElementsByTagName(...)
built-in method returns an HTMLCollection
of any element(s) that have a specified tag, i.e.:
getElementsByTagName('h1')
would return a collection of all<h1>
tagsgetElementsByTagName('h1 h2')
would return a collection of all<h1>
and<h2>
tags
Pertaining to the getElementsByClassName
and getElementsByTagName
method, an HTMLCollection
has a typeof
value equal to object
but behaves like an array. That is, we can access values with indices.
For example, for the following HTML:
<html>
<head></head>
<body>
<div>
<a class="navlink" href="#">Link 1</a>
<a class="navlink" href="#">Link 2</a>
</div>
</body>
</html>
We can access each anchor tag using array-like notation (by the way, the innerHTML
property allows us to access the content of the tag):
const navLinks = document.getElementsByClassName('navlink')
console.log(navLinks[0].innerHTML)
// "Link 1"
console.log(navLinks[1].innerHTML)
// "Link 2"
The querySelector
method
We can access a DOM element using a CSS-style selector with the querySelector
method:
- with
document
we would call thequerySelector
method - we then pass a CSS selector as an argument to that method
- assigning the method call to a variable, the method will return an
Element
representing the first element that matches the CSS selector- if it finds no matches, then it returns
null
- if it finds no matches, then it returns
let allToAnchor = document.querySelector("#someAnchor")
let allToClass = document.querySelector("a.important")
let allToTagList = document.querySelector("ul")
let allLinksToJon = document.querySelector("a[href='https://jxc.ca']")
let general = document.querySelector(selector)
// the *first* HTML Element matching the selector
The querySelectorAll
method
We can also access a selection of DOM elements that match a CSS-style selector with the querySelectorAll
method:
- with
document
we call thequerySelectorAll
method - we then pass a CSS selector as an argument to that method
- assigning the method call to a variable, the method will return a
NodeList
- elements will arrange in the order that they appear in the HTML document, with indices
- if it finds no matches, then it will return an empty
NodeList
let allToAnchor = document.querySelectorAll("#someAnchor")
let allToClass = document.querySelectorAll("a.important")
let allToTagList = document.querySelectorAll("ul")
let allLinksToJon = document.querySelectorAll("a[href='https://jxc.ca']")
let general = document.querySelectorAll(selector)
// *all* HTML Elements matching the selector
Like the getElement...
methods, we can access each result with array-like notation. For example, with an HTML of:
<html>
<body>
<div>
<p class="navlink">This won't query because it's a paragraph</p>
<a class="navlink" href="#">Link 1</a>
<a class="navlink" href="#">Link 2</a>
</div>
</body>
</html>
We can access the anchor (a
) tags with a class name of navlink
like such:
const aNavLinks = document.querySelectorAll('a.navlink')
console.log(aNavLinks[0].innerHTML)
// "Link 1"
console.log(aNavLinks[1].innerHTML)
// "Link 2"
Note how we specified a.navlink
and not just navlink
in the "query", so that the aNavLinks
variable won't include the paragraph with the class name of navlink
!
DOM traversal
As we might know already, the DOM is structured like a family tree of HTML tags. Each tag (except the <html>
tag) has a parent and, in all non-trivial cases, the <html>
tag has children.
Thus, JavaScript has methods which allow us to access "parent" and "children" nodes of each tag. This allows us to "traverse" or travel through the DOM tree.
The parentNode
property
When we call a querySelector
method, we can simply chain the parentNode
property to access the result's parent node:
let objectsParent = document
.querySelector('.object')
.parentNode
A very universal example of this:
let objectsParent = document
.querySelector('body')
.parentNode
which would yield the <html>
tag node!
The children
property
When we call a querySelector
method, we can simply chain the children
property to access an HTMLCollection
of the result's children:
let objectsChildren = document
.querySelector('.object')
.children
A very universal example of this:
let objectsChildren = document
.querySelector('body')
.children
...which should yield an HTMLCollection
of the DOM representation of the <head>
and <body>
tags!
The firstChild
property
We can also just get the first child of each object with firstChild
:
let objectsFirstborn = document
.querySelector('.object')
.firstChild